配置 rollup
什么是rollup
是一个 JavaScript 模块打包工具,可以将多个小的代码片段编译为完整的库和应用。它使用的是ES6版本的模块标准ES Module,只打包 js ,打包速度快,打包生成的包体积小
开发框架/ 组件库的时候使用 Rollup 更合适
首先在 src 下创建 lib 文件夹 , 然后创建 index.ts , 将所有需要导出的组件导出
组件库示例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| export { default as Alert } from '../components/Alert/Alert.vue'
export { default as Button } from '../components/Button/Button.vue' export { default as ButtonGroup } from '../components/ButtonGroup/ButtonGroup.vue' export { default as Collapse } from '../components/Collapse/Collapse.vue' export { default as Common } from '../components/Alert/Alert.vue'
export { default as Container } from '../components/Container/Container.vue' export { default as DatePicker } from '../components/DatePicker/DatePicker.vue'
export { default as Dropdown } from '../components/Dropdown/Dropdown.vue' export { default as Form } from '../components/Form/Form.vue' export { default as Icon } from '../components/Icon/Icon.vue' export { default as Input } from '../components/Input/Input.vue' export { default as Link } from '../components/Link/Link.vue' export { default as Message } from '../components/Message/Message.vue' export { default as MessageBox } from '../components/MessageBox/MessageBox.vue' export { default as Rate } from '../components/Rate/Rate.vue' export { default as Select } from '../components/Select/Select.vue' export { default as Switch } from '../components/Switch/Switch.vue' export { default as Tooltip } from '../components/Tooltip/Tooltip.vue'
|
然后配置 rollup.config.js
首先安装以下插件
rollup-plugin-esbuild
将ts编译成ES6
@vitejs/plugin-vue
rollup-plugin-scss
转译scss文件,配合DartSass
使用
rollup-plugin-terser
代码压缩插件,转译es6+语法
在根目录新建一个 rollup.config.js
文件,写入以下配置
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
|
import esbuild from 'rollup-plugin-esbuild' import vue from '@vitejs/plugin-vue' import scss from 'rollup-plugin-scss' import dartSass from 'sass' import { terser } from 'rollup-plugin-terser'
export default { input: 'src/lib/index.ts', output: { globals: { vue: 'Vue', }, name: 'Weirdo-UI', file: 'dist/lib/weirdo-ui.js', format: 'umd', plugins: [terser()], }, plugins: [ scss({ include: /.scss$/, sass: dartSass }), vue({ include: /.vue$/, }), esbuild({ include: /.[jt]s$/, minify: process.env.NODE_ENV === 'production', target: 'es2015', }), ], }
|
rollup 包安装的问题
请先全局安装 rollup
你可能会遇到问题

执行 pnpm setup
, 自动设置环境变量 (自动创建全局二进制文件目录。该命令会设置全局的 bin 目录,并将其添加到系统的 PATH 环境变量中)

然后我们只需设置 pnpm 的全局 bin 路径 为你安装node的地方
如何查看呢?
where node
然后我们只需设置pnpm的源即可
(手动设置 pnpm 的 global-bin-dir
配置,将全局二进制文件目录指定为一个存在的目录)

然后执行 rollup -c
打包文件如下

npm包上传
package.json
添加如下配置
"weirdo-ui",1 2 3 4 5 6
| "name": "weirdo-ui", "version": "0.0.1", "files": [ "dist/lib/*" ], "main": "dist/lib/weirdo-ui.js",
|
其中files字段是上一步rollup打包的文件,也是用户安装你的软件包时会下载的文件
main字段是项目主文件,假设项目名name为foo
,用户使用require("foo")
,即可引入该主文件的module.exports对象
注意 : 如果你的package.json 中有 private 字段, 请删除, 否则在发布时会报这个错误

在执行pnpm publish
之前,保证没有使用淘宝源
1 2 3
| pnpm config get registry
pnpm config set registry registry.npmjs.org/
|
发布包
1 2 3
| pnpm login
pnpm publish
|
之后每次发布新版本之前要记得修改 版本号
使用组件库
App.vue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| <script setup lang="ts"> import {Button, Rate} from 'weirdo-ui' </script>
<template> <div> <Button type="success" plain> Primary </Button> <Button type="success" plain> Success </Button> <Button type="warning" plain> Warning </Button> <Button type="danger" plain> Danger </Button> <Button type="info" plain> Info </Button> </div> <Rate :max="10" color="pink"></Rate> </template>
<style scoped></style>
|
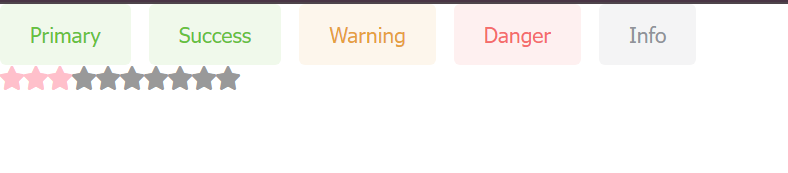
https://2weirdo.github.io/weirdo-ui/ 这是我的组件库, 欢迎 star ~ 持续更新
参考文章
- https://juejin.cn/post/7198506378432200761
- https://juejin.cn/post/7043399990382690334?from=search-suggest#heading-0